Getting Started
Record your Playwright tests
Create a new Test Suite team
Start by visiting our new test suite form. It will create an API key and guide you through each step.
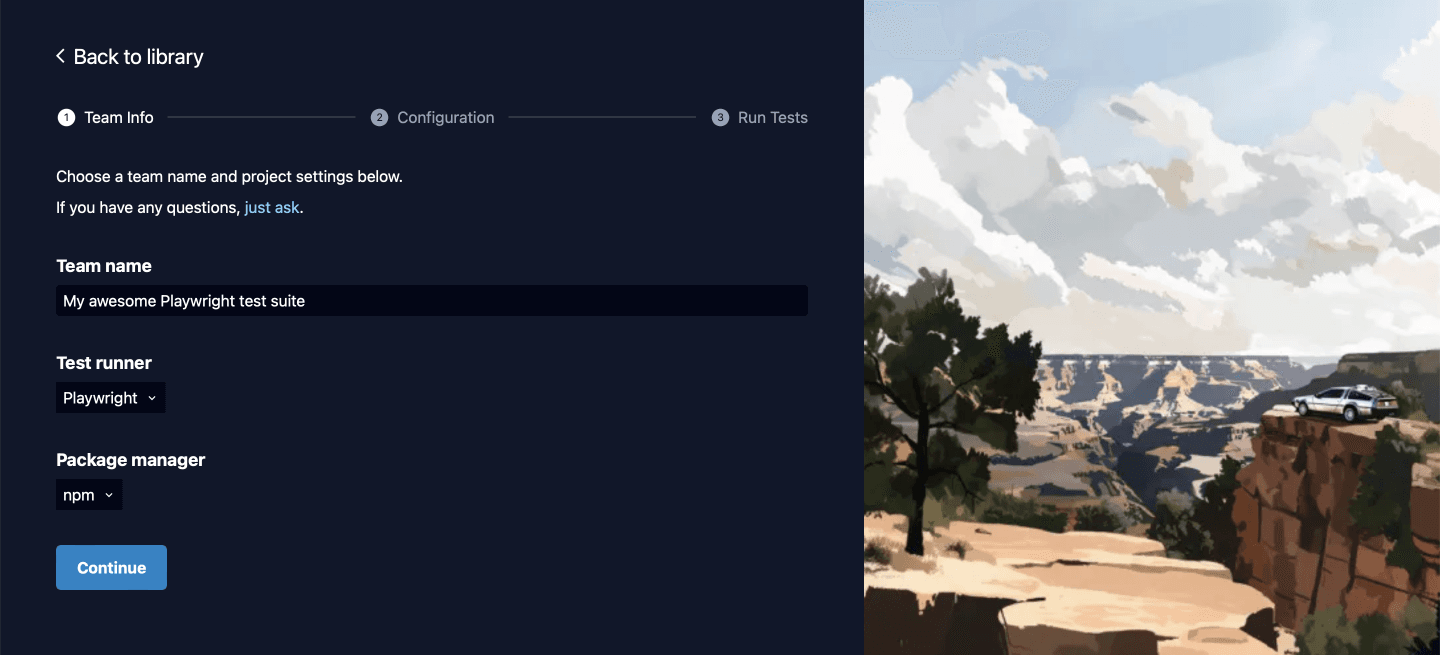
Install the Replay browser
Terminal
npx replayio install
Save your API key
To use your API key, you can either use dotenv package and save it to a .env
file or add the API key to your environment directly.
.env
REPLAY_API_KEY=<your_api_key>
Update playwright.config.js
playwright.config.ts
1import { PlaywrightTestConfig, devices } from "@playwright/test";2import {3 devices as replayDevices,4 replayReporter5} from "@replayio/playwright";6import "dotenv/config";78const config: PlaywrightTestConfig = {9 reporter: [10 replayReporter({11 apiKey: process.env.REPLAY_API_KEY,12 upload: true,13 }),14 ["line"],15 ],16 projects: [17 {18 name: "replay-chromium",19 use: { ...replayDevices["Replay Chromium"] },20 },21 ],22};23export default config;
Record your test
With everything set up, you can now run playwright test
to record and upload your first Playwright replays.
Terminal
npx playwright test --project replay-chromium
Terminal
➜ npx playwright testRunning 1 test using 1 worker[1/1] things-app.spec.ts:14:7 › Todos › should allow me to add todo items[replay.io]: 🕑 Completing some outstanding work ...[replay.io]:[replay.io]: 🚀 Successfully uploaded 1 recordings:[replay.io]:[replay.io]: ✅ should allow me to add todo items1 passed (2.1s)
Check out this replay for a detailed walkthrough on debugging a flaky Playwright test.
Record your test suite in CI
Now that you're ready to inspect your local tests, the next step is to record your tests in CI. Learn how to set up Replay with your Playwright tests on GitHub Actions and other CI providers.
Learn how to record your tests, manage your test suite and debug flaky tests using Replay DevTools
Record Your CI Test Run
Learn how to integrate Replay into your Continuous integration service
Replay DevTools
Learn how to use Replay DevTools to debug your tests.
Test Suite Management
Test Suite Dashboard helps you stay on top of your test suite health.
Debugging tips
Learn about how to effectively debug flaky or failing tests